API
Filestash has a builtin API that allows developers to interact with any of the supported storage in a consistent manner, whether it’s S3, SFTP, FTP, .... To use it, you first need to generate an API key from the admin console:
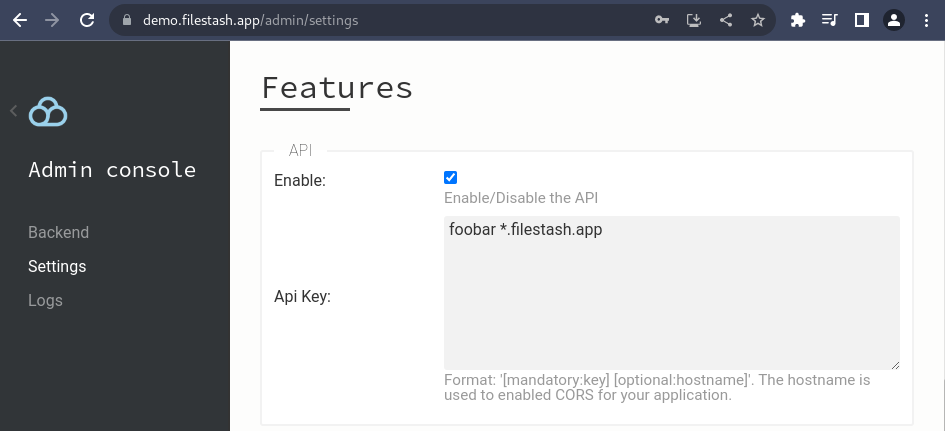
We've setup the "foobar" api key and have associated some cors rules for any filestash subdomain effectively making this page entirely interactive. Once done, we've made a shared link called 'shareID' which we will also be using to interact with the API. If you want to copy paste the curl commands scattered in this page, you will need to do a bit of setup:
export INSTANCE=https://demo.filestash.app export SHARE=shareID export KEY=foobar
API for File management
There's an API endpoint for every possible file management operation you can think of:
- list files
- upload a file
- download a file
- zip something
- rename something
- delete something
- create a directory
- create an empty file
curl "$INSTANCE/api/files/ls?share=$SHARE&key=$KEY&path=/"
{ "status": "ok", "results": [ { "name": "README.org", "type": "file", "size": 281, "time": 1591432761000 }, { "name": "Documents", "type": "directory", "size": 0, "time": 1591432774000 } ] }
curl "$INSTANCE/api/files/cat?share=$SHARE&key=$KEY&path=/input.txt" \ -X POST --data @input.txt
curl -X GET "$INSTANCE/api/files/cat?key=$KEY&share=$SHARE&path=/input.txt"
curl "$INSTANCE/api/files/zip?share=$SHARE&key=$KEY&path=/input.txt"
curl -X POST "$INSTANCE/api/files/mkdir?key=$KEY&share=$SHARE&path=/tmp/"
curl -X POST "$INSTANCE/api/files/touch?key=$KEY&share=$SHARE&path=/test.txt"
curl -X POST "$INSTANCE/api/files/mv?key=$KEY&share=$SHARE&from=/input.txt&to=/test/input.txt"
curl -X POST "$INSTANCE/api/files/rm?key=$KEY&share=$SHARE&path=/test/"
If you want to create shared links programmatically, check this out